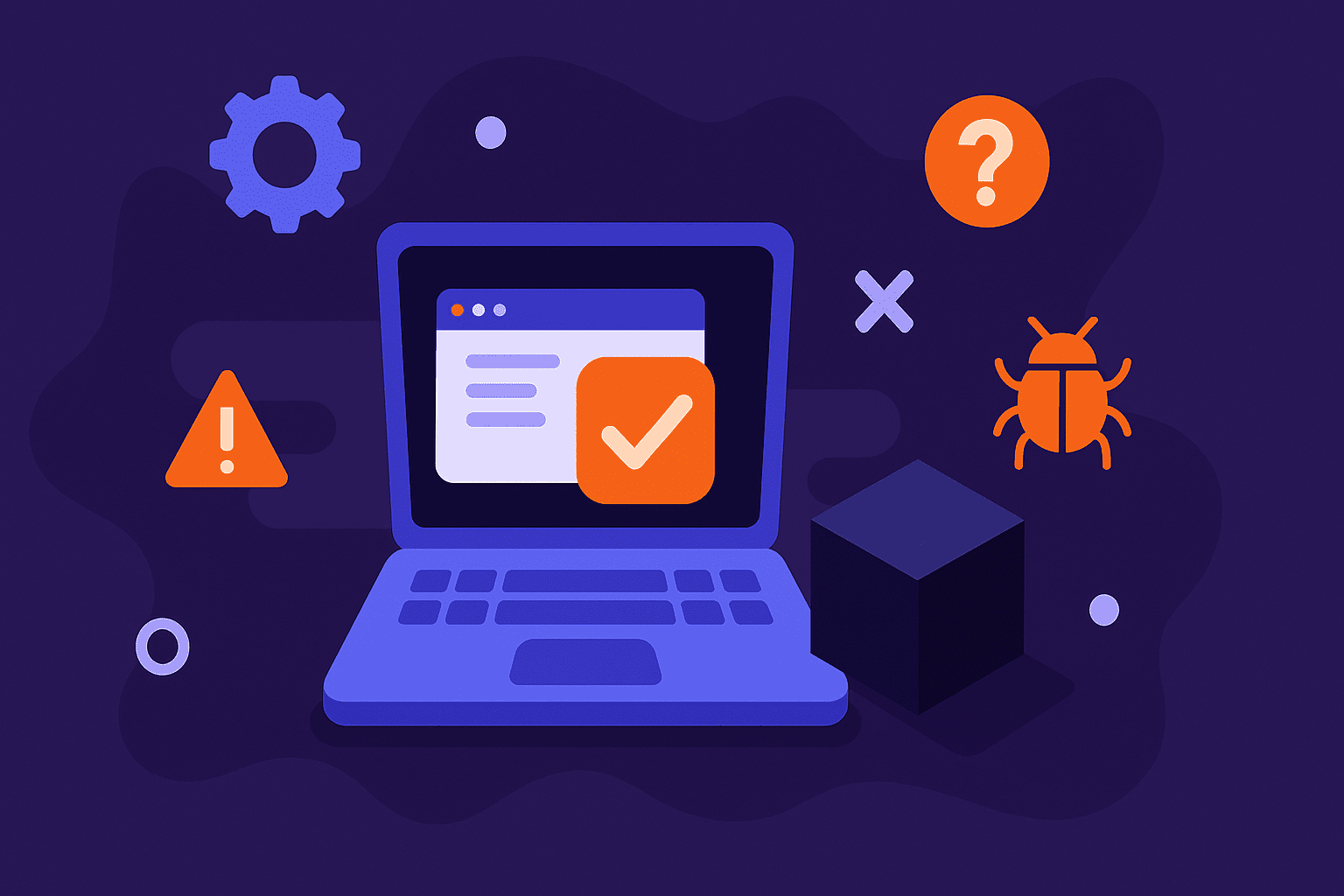
Building a successful mobile or web app requires more than just good code—it also depends on the right tools.
One of the most essential tools for developers is the software development kit (SDK).
But what exactly is an SDK, and why is it so important in app development?
In this article, we’ll break down the essentials and show how SDKs can help you build better apps faster.
Table of Contents
What is an SDK?
Imagine the building blocks and tools needed to construct a house.
Even if you know how to build it, you wouldn’t start entirely from scratch—you’d use prefabricated materials like bricks, wood, and fixtures.
Similarly, SDKs provide developers with ready-made tools and components to build mobile, web, and desktop applications more efficiently.
Here’s how the Cambridge Dictionary defines an SDK:
Now that we’ve defined SDKs, let’s explore their common components.
Common SDK components
An SDK typically includes several key components that help developers streamline the app development process.
The first is code libraries.
These are collections of pre-written code designed to handle common tasks within an app, such as user authentication, data storage, or performance analytics.
Rather than writing this code from scratch, developers can integrate these libraries into their apps to save time and effort.
For example, an SDK’s library may include code for integrating a login system or tracking user behavior, which is often crucial for building personalized experiences.
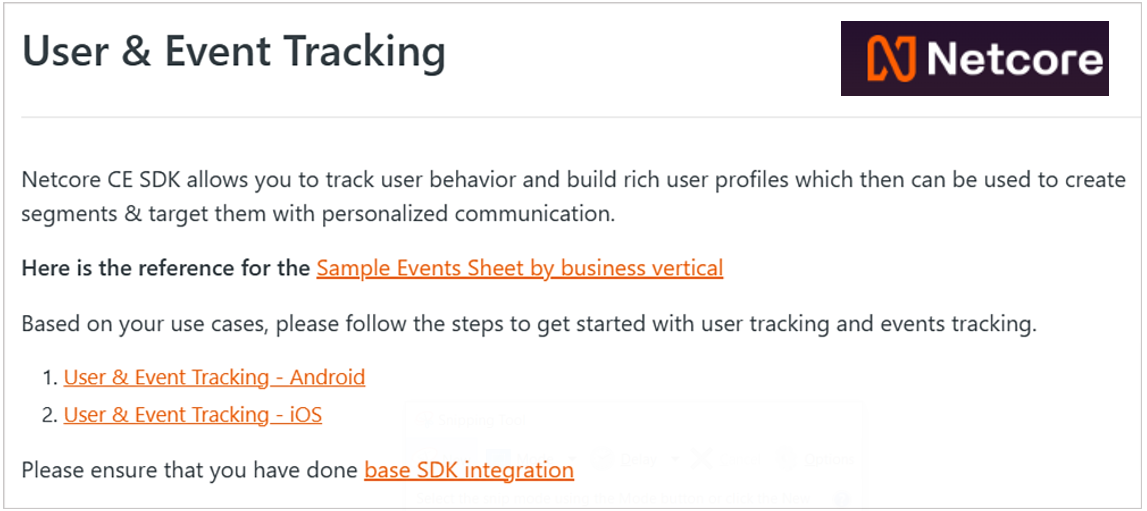
The second common SDK component is tools.
They help developers build robust, error-free applications by streamlining development and troubleshooting processes.
Typically, they include:
debugging utilities | to identify and fix code issues |
testing environments | for running simulations and verifying app behavior |
performance profilers | to measure how efficiently the app runs |
compilers | to convert source code into executable files |
The next SDK component is application programming interfaces (APIs).
APIs work closely with libraries and tools by enabling an app to interact with its underlying platform or external services.
They define how different software components communicate, allowing your app to access platform-specific or third-party features like location services or push notifications.
While APIs are integral components of many SDKs, the reverse never happens.
In other words, an entire SDK can’t—by definition—be contained within an API.
However, like SDKs, APIs have rules, protocols, and documentation to guide developers through proper implementation.
For example, the Stripe payment SDK includes APIs designed to help developers integrate payment processing into their apps.
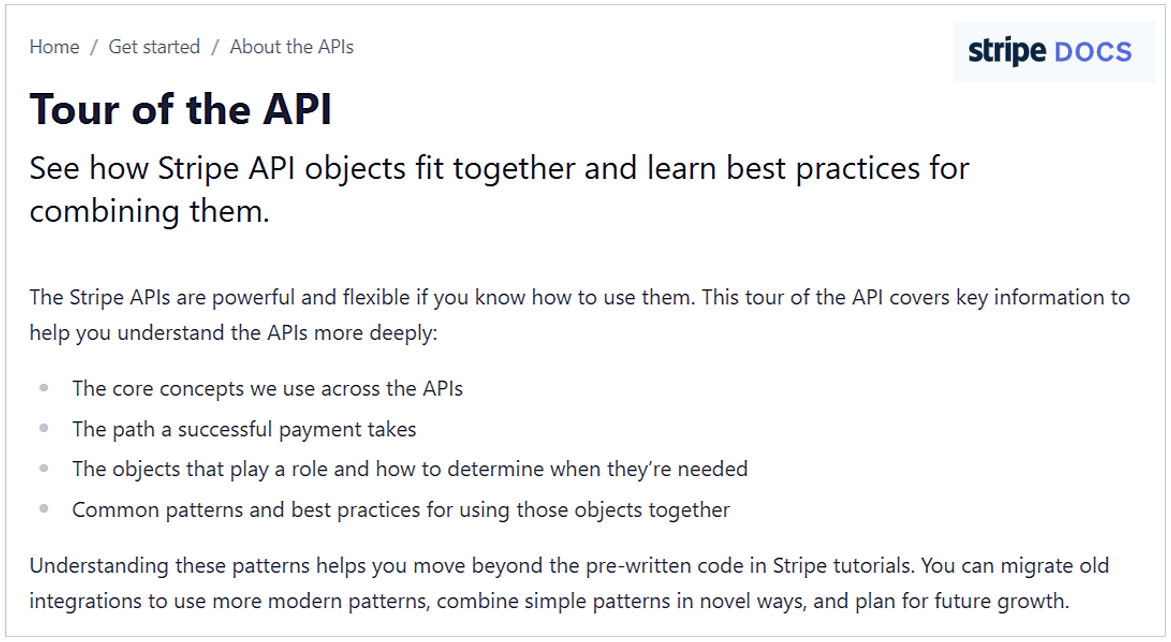
Next, SDKs often include pre-designed user interface (UI) elements that help developers build consistent and user-friendly apps.
These elements can include buttons, sliders, and navigation bars—all tailored to the native platform to ensure a seamless user experience (UX) across different devices and applications.
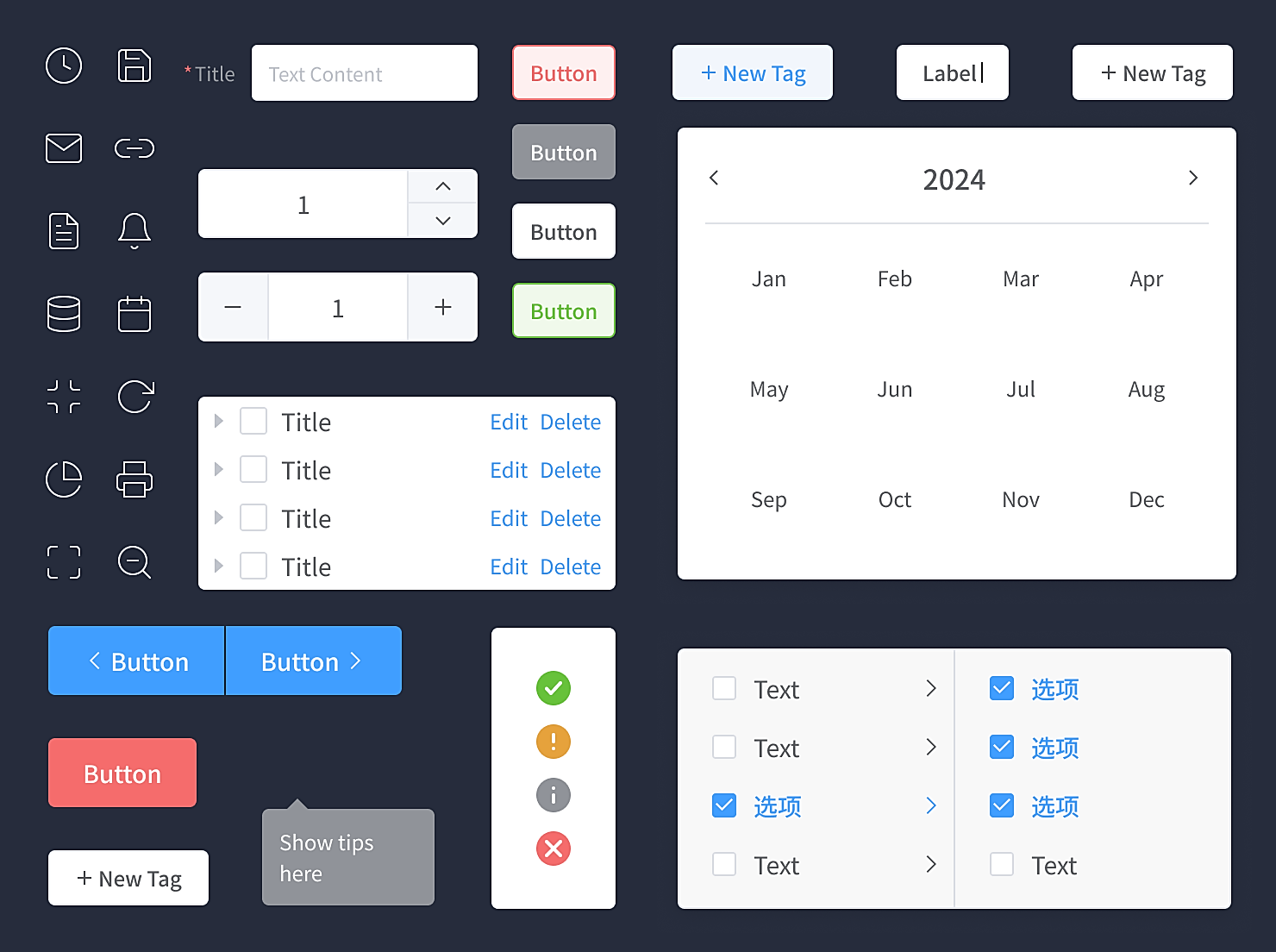
These pre-built UI components are usually found in the SDK’s libraries as ready-to-use code blocks or functions that developers can easily add and customize in their apps.
And this brings us to the last SDK component—documentation.
Comprehensive documentation is crucial for helping developers incorporate an SDK into their Integrated Development Environment (IDE) and, ultimately, into their app.
This documentation typically includes detailed guides, tutorials, and code samples or templates.
With good documentation, developers can easily understand how to use the SDK components and integrate them into their projects.
With the common SDK components covered, let’s explore the different types of SDKs.

Get unreal data to fix real issues in your app & web.
Types of software development kits
There’s certainly no shortage of SDK types, reflecting the wide range of applications and technologies they support.
We’ll focus on some of the most widely used SDK types.
The first major group is platform-specific SDKs—designed for specific platforms or operating systems.
It can be further divided into:
- mobile SDKs like the Android SDK and iOS SDK, used to build mobile apps tailored for native platforms,
- web SDKs like the Facebook SDK or Slack SDK, designed for developing web applications,
- desktop SDKs like the Windows SDK or macOS SDK, focused on developing apps for desktop environments.
Next, there are cross-platform SDKs, which enable developers to create apps that run on multiple platforms (e.g., iOS, Android, web) using a single codebase.
Popular examples include frameworks like React Native and Flutter.
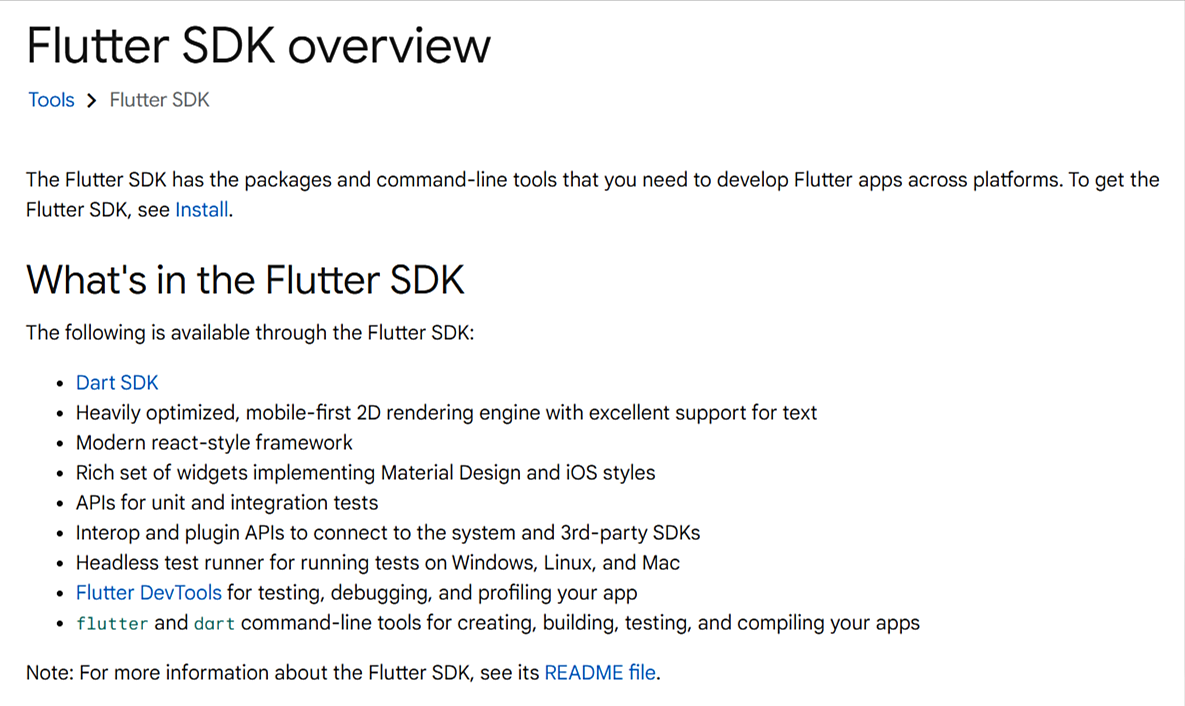
When cross-platform SDKs are used, they often rely on platform-specific SDKs behind the scenes to ensure compatibility with each target platform.
Another important type is programming language SDKs, which are designed for specific programming environments, such as the .NET SDK or Python SDK.
Since these SDKs help developers build apps in a particular programming language, they often come in various versions, each tailored for different purposes and services.
For instance, the Python SDK shown below enables developers to manage permissions and roles within their apps.
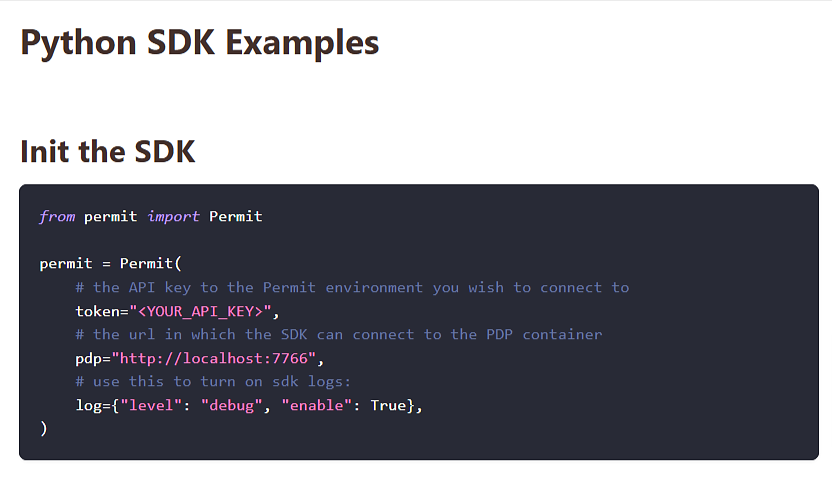
While the above SDK types cover the major categories, there are also many specialty SDKs tailored to specific functionalities.
These function-specific SDKs are typically chosen based on their compatibility with developers’ primary SDK (e.g., Android SDK, or React Native SDK).
Common types of specialty SDKs include:
- authentication SDKs
- messaging SDKs
- location mapping SDKs
- payment SDKs
- analytics SDKs, and more
For example, payment SDKs that developers use to set up secure payment processing in their apps.
Think Stripe SDK and PayPal SDK.
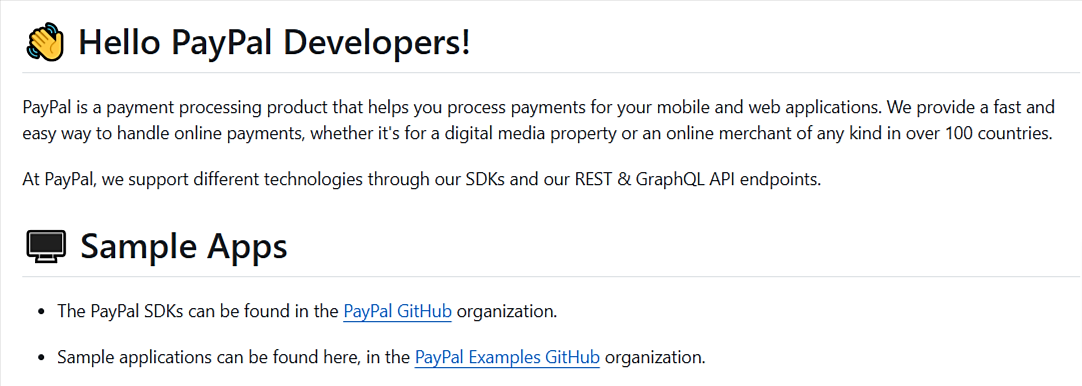
Another important group is analytics SDKs, which enable developers to track app performance and user behavior.
Popular examples include the Firebase Analytics SDK, Mixpanel SDK, and others.
By integrating an analytics SDK into their app, developers can collect and analyze valuable data on user interactions and app performance.
The next prominent group is bug-reporting SDKs.
These SDKs provide developers with key information needed to fix bugs and crashes during app development and post-release.
A good example of a bug-reporting SDK is our Shake.
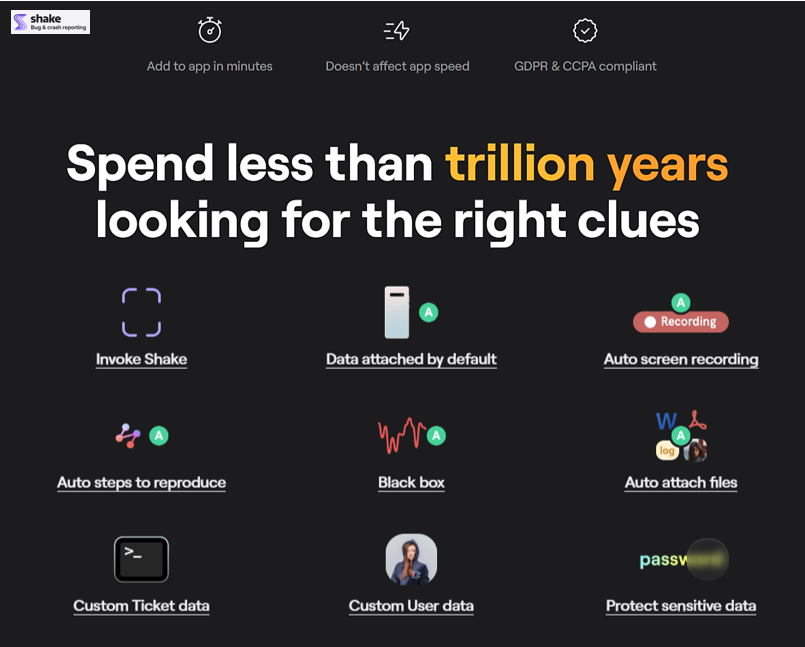
After the Shake SDK is added to your app, users can simply shake their phone—or use another method—to report an issue and optionally provide feedback.
This will generate a report (ticket) that automatically includes over 70 data points, providing developers with detailed context to address the issue.
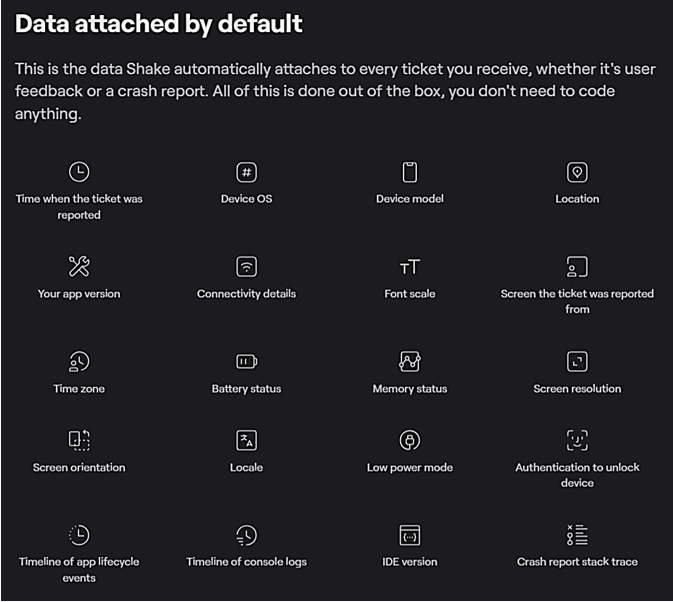
By delivering detailed data and insights, bug-reporting SDKs enable developers to diagnose and fix bugs more efficiently—before they escalate.
In addition to Shake, other popular bug-reporting SDKs include Firebase Crashlytics, BugSnag, Instabug, and others.
As we’ve explored at least some major SDK types, let’s now see what makes a good SDK.
What makes a good SDK?
One critical factor that makes an SDK truly effective is the ease of integration.
A great SDK should offer a straightforward setup process and seamless compatibility with major platforms.
For example, the Shake SDK can be added to your mobile or web app in just minutes.
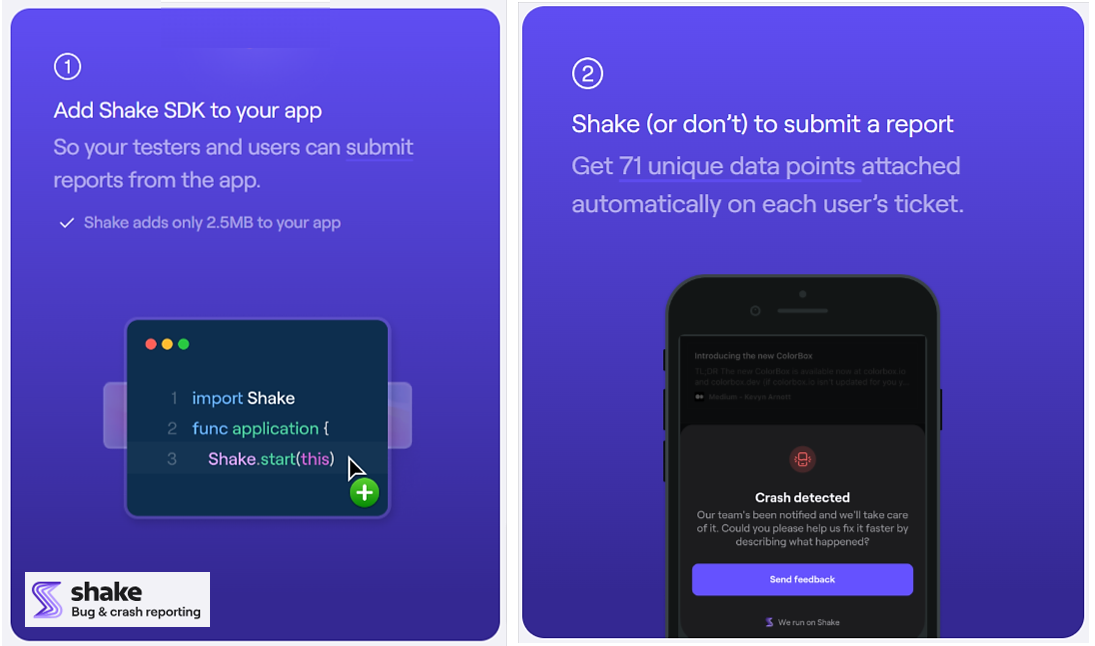
As shown, the Shake SDK has a small footprint—only 2.5 MB—ensuring it won’t affect your app’s performance.
This leads to the next essential quality: performance efficiency.
An SDK must be lightweight and optimized to minimize its impact on app speed, memory usage, and battery life.
Another critical feature of a great SDK is comprehensive documentation.
Clear, thorough documentation is vital for guiding developers through setup, usage, and troubleshooting.
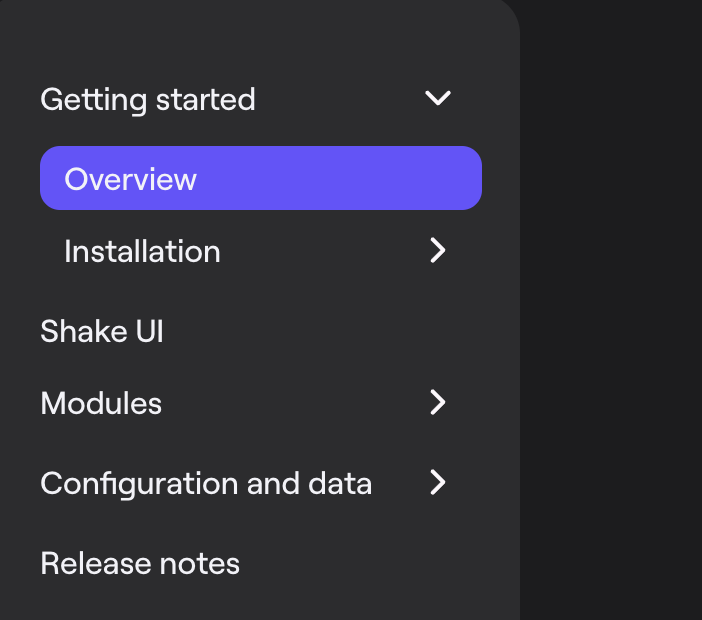
Next is the non-negotiable factor of security.
A reliable SDK must offer data encryption, secure authentication, and compliance with data protection standards like GDPR and CCPA.
Lastly, community support and regular updates are crucial.
Access to forums, user groups, and timely updates ensures developers can quickly resolve issues and benefit from continuous improvements.
Together, these qualities make an SDK not just functional but a truly valuable tool for developers—let’s see how it works.
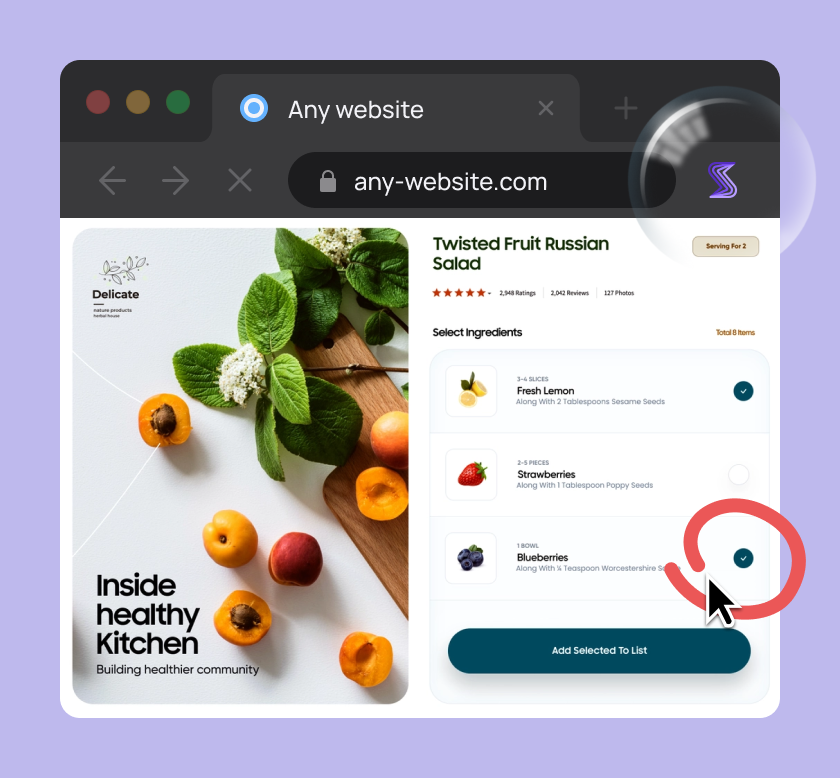
Capture, Annotate & Share in Seconds with our Free Chrome Extension!
How does an SDK work?
When developers want to integrate an SDK into their app, they typically follow a series of steps to ensure everything is set up correctly.
This process starts with downloading and installing the SDK within their preferred Integrated Development Environment (IDE), such as Android Studio, Xcode, Visual Studio, and others.
Next, developers configure the SDK by following its documentation, which typically includes clear instructions for:
- adding dependencies,
- setting permissions, and
- initializing the SDK within the project.
Once integrated, developers can use the SDK’s tools, functions, or APIs to implement specific features or functionalities in the app.
For example, let’s say a developer wants to embed Google Maps into their Android app.
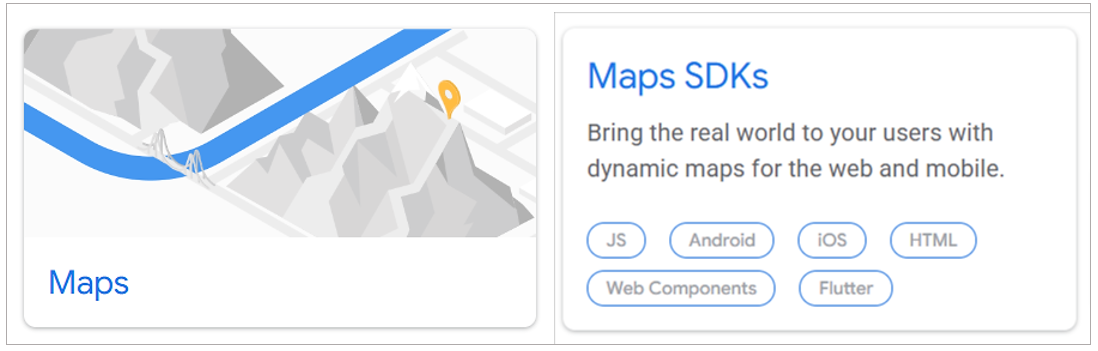
They would first create a new project in Android Studio or another suitable IDE.
Then, they would access Google Cloud Console, click on “Enable APIs and Services,” and search for “Maps SDK for Android.”
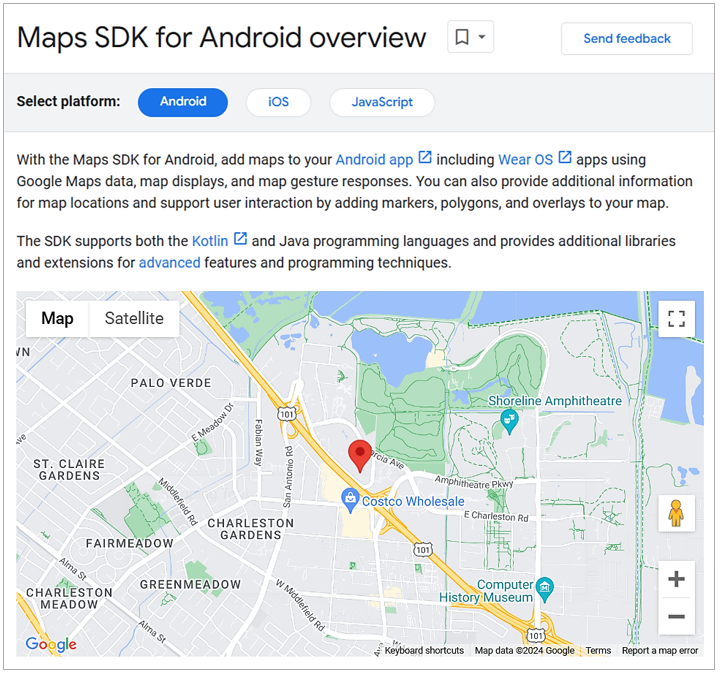
After enabling the Maps SDK, they would create credentials to generate an API key.
This step is followed by:
- adding the necessary Google Maps dependencies to the project’s file,
- configuring the API key in the Android Manifest, and
- adding a map fragment to the app’s layout.
They would then initialize the map in their activity or fragment code using the Maps API to load and customize the map view.
After completing these steps, the developer can launch a digital emulator or connect a physical Android device to test the new map feature.
If everything works smoothly, the app is ready for release with fully integrated Google Maps functionality.
This streamlined process demonstrates how SDKs simplify complex feature integration.
Next, let’s explore how SDKs help developers accelerate development and improve app performance.
How do SDKs help?
Instead of building complex features from scratch, developers can rely on tried-and-tested tools and libraries to handle repetitive or time-consuming coding tasks.
Therefore, a faster development process is one of the most significant advantages of using SDKs.
For example, securely developing a payment system could take weeks—but using a trusted payment SDK like Stripe can reduce that time to just a few hours.
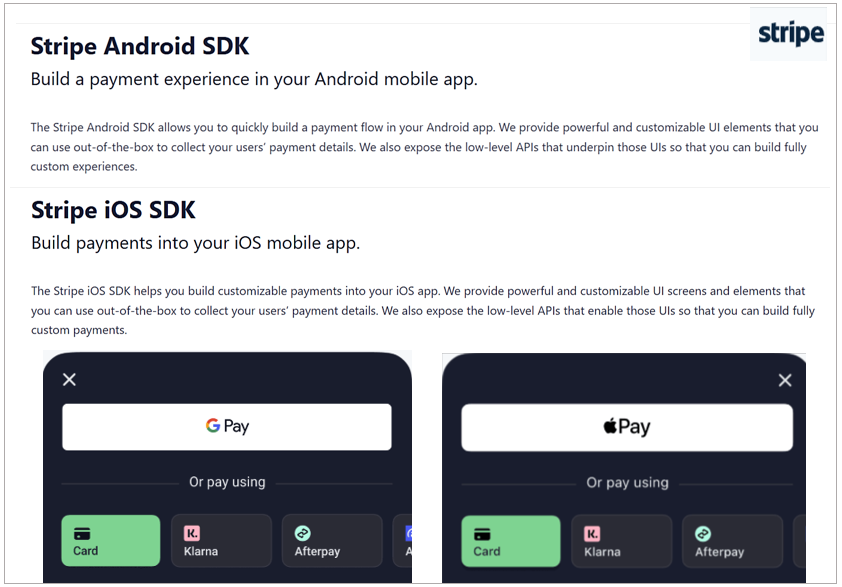
Beyond saving time, SDKs also enhance app functionality by giving developers access to advanced tools and technologies that would otherwise be difficult to implement.
Many modern apps rely on third-party services for features like:
- authentication (e.g., Google Sign-in),
- analytics (e.g., Firebase Analytics), and
- payment processing (e.g., Stripe).
SDKs simplify integrating these services, making them essential for developers aiming to implement complex features efficiently.
Another great example is adding machine learning (ML) and artificial intelligence (AI) functionalities to apps.
This can be incredibly complex, but SDKs like Google’s LiteRT (formerly TensorFlow Lite) allow developers to integrate AI features without needing deep AI expertise.
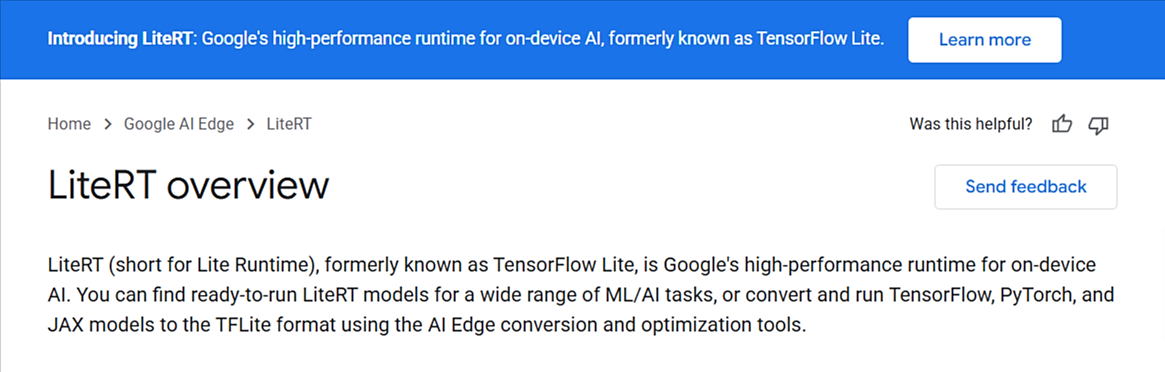
Standardization is another major benefit of using SDKs.
SDKs offer consistent methods and structures for implementing features, ensuring uniformity across different apps.
This results in cleaner, more organized code, making apps easier to maintain and scale.
Standardization also ensures that features work reliably across various devices and platforms.
An added advantage is error reduction.
Since SDKs are built, tested, and maintained by specialized teams and scrutinized by developer communities, they typically provide reliable, well-optimized code.
This minimizes bugs and simplifies debugging, allowing developers to focus on building their core product instead of troubleshooting technical issues.
Still, bugs and crashes are inevitable, but SDKs like Shake make it easier for developers to quickly identify and resolve these issues.
Given all these advantages, it’s clear why SDKs are essential tools for creating efficient, reliable, and high-performing apps.
Conclusion
With all this information behind us, it’s clear that SDKs provide developers with essential components, tools, and documentation for efficient app development.
They enable developers to implement complex features more effectively, enhance app performance, and minimize errors.
By leveraging the right SDKs, your dev team can focus on creating innovative, high-quality apps while saving time and resources.