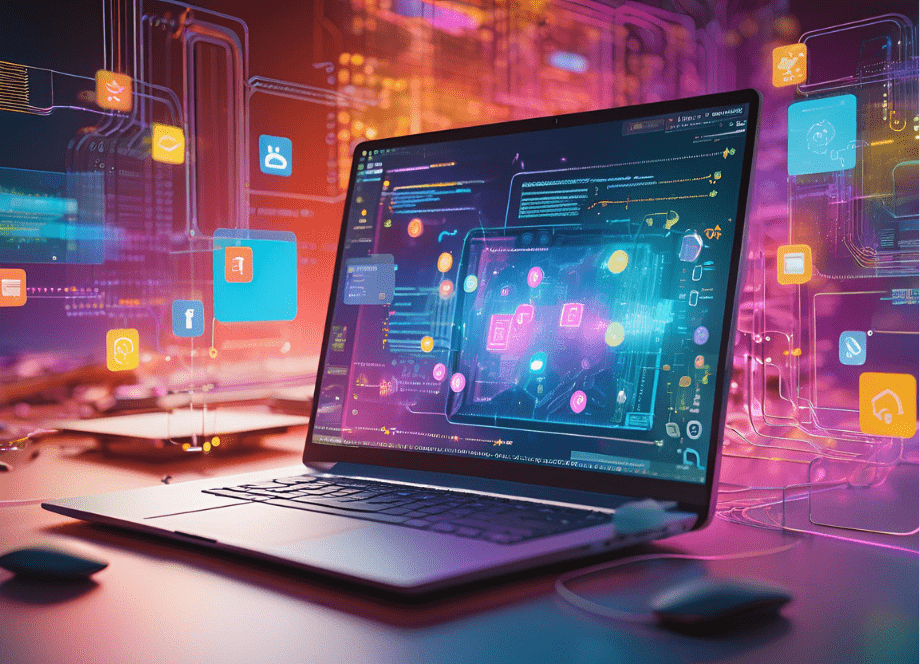
So, you’ve created a powerful feature or service, and now you want to design an SDK to encourage developers to integrate it into their apps.
A well-crafted SDK can make adoption seamless and offer a smooth integration experience.
Conversely, a poorly designed one can push developers away.
The easier your SDK is to use, the more likely it will become a go-to tool in their tech stack, whether they are development agencies or freelancers.
On that note, let’s explore seven best practices for building intuitive, efficient, and developer-friendly SDKs.
Table of Contents
Prioritize simplicity in setup and installation
To use an SDK, developers must first install it and then configure it for their specific development environment.
This is often the most crucial interaction developers have with your SDK, and that’s why it’s critical to make it effortless.
If the installation is complicated or unclear, it can lead to frustration, and developers might abandon the integration altogether.
This comment from Stack Overflow illustrates a developer’s frustrating experience with trying to install the .NET SDK with Visual Studio.
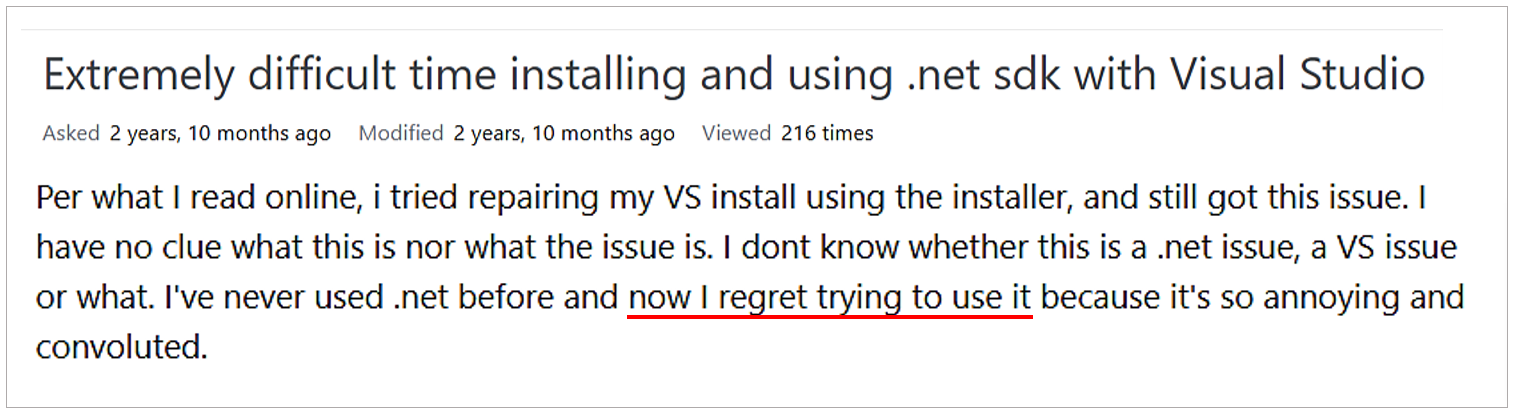
To prevent this with your SDK, you should address common pain points developers face during installation and setup.
These typically include:
- complex installation procedures,
- confusion over setup steps,
- unclear error messages, and
- conflicting dependencies.
One of the most effective ways to simplify the installation process is to leverage package managers.
These tools automatically handle dependencies, significantly reducing installation complexity.
Package managers can be standalone tools like npm (Node Package Manager) or integrated into popular build tools such as Gradle for Android and Swift for iOS.
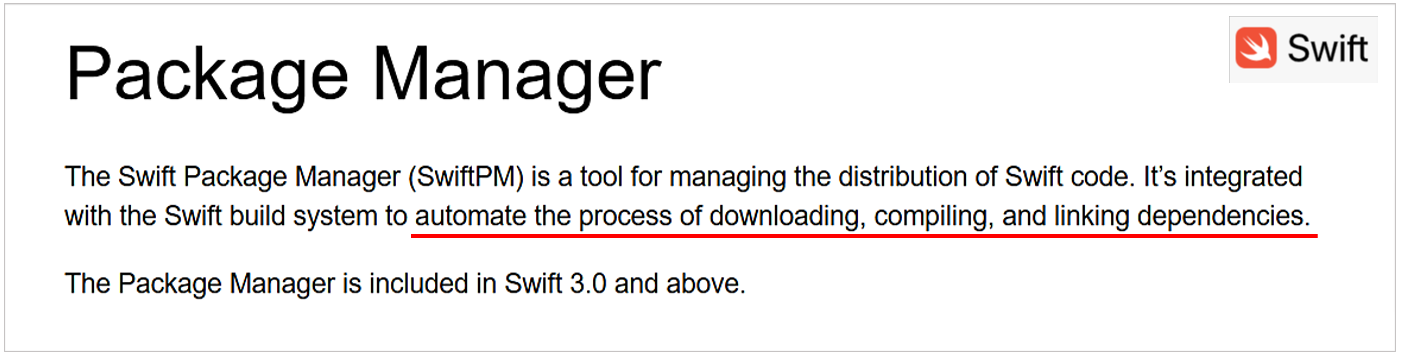
By streamlining installation, version control, and dependency management, package managers eliminate many manual setup tasks that often cause confusion and errors.
Dedicated installation packages with automatic dependency resolution allow developers to integrate your SDK quickly—without manual configuration or troubleshooting.
Ultimately, a seamless installation followed by an intuitive setup process improves developer satisfaction and drives greater adoption, usability, and compatibility of your SDK.
Provide comprehensive documentation
Easy installation and setup are only the first step.
Developers also need clear, detailed documentation to understand how to use your SDK to its full potential.
Comprehensive documentation typically includes:
- step-by-step guidance on how to install and configure the SDK,
- code examples demonstrating effective usage, and
- a well-organized, searchable structure for easy navigation.
Shake, our bug-reporting SDK, exemplifies these principles.
Its documentation goes beyond clear installation steps to provide quick guides and practical examples, helping developers integrate the SDK seamlessly into their apps.
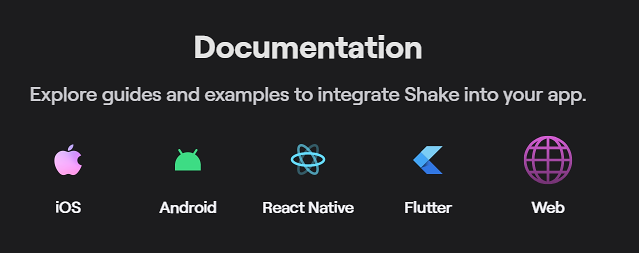
As shown, documentation is organized by platform, allowing developers to click on icons for platform-specific guides.
These guides detail installation, setup, and advanced use cases.
Here’s an example for React Native.
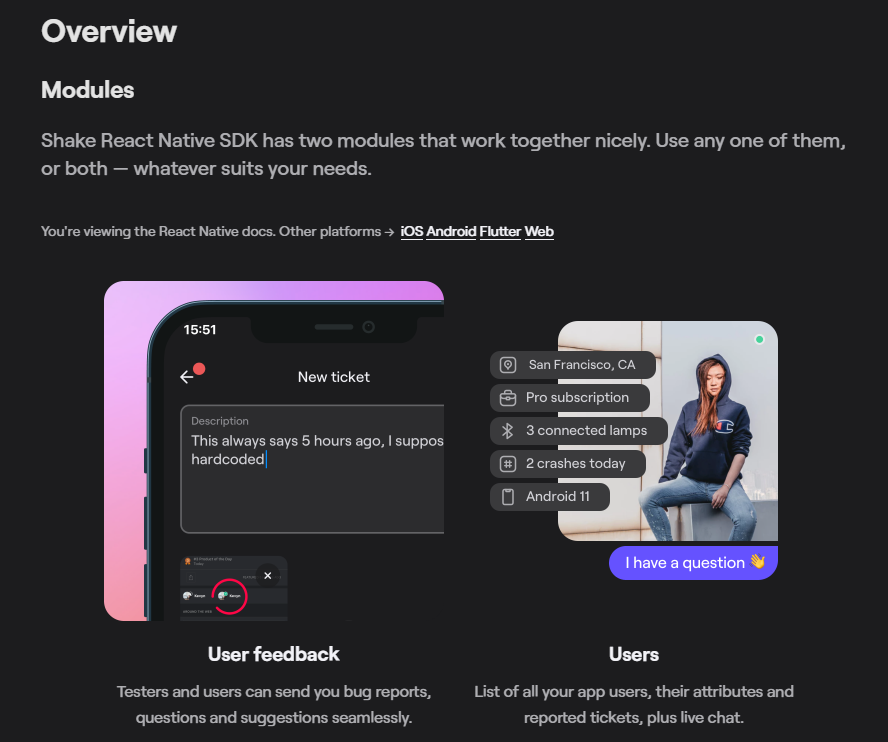
This explains that Shake works through two core modules, of which you can use both or just the one that fits your bug-reporting needs.
The “User feedback” module enables testers and developers to automatically generate detailed bug reports and, optionally, give feedback.
The “Users” module gives you a list of all app users and their reported tickets, also enabling you to live chat with users.
Shake’s documentation also includes minimum installation requirements and ensures developers always have access to the latest SDK version.
This thoughtful, developer-friendly approach reduces friction, promotes adoption, and demonstrates how comprehensive documentation can drive the success of your SDK.

Get unreal data to fix real issues in your app & web.
Design APIs that feel intuitive to use
APIs, or Application Programming Interfaces, are the bridge between your SDK and the developers using it.
They define how developers interact with your SDK’s functionality, making them a critical part of the SDK design process.
A well-designed API ensures that developers can easily integrate and use your SDK’s features in their apps without unnecessary complexity.
In the words of Nathan Darst, Director of SDK Engineering for Kochava:
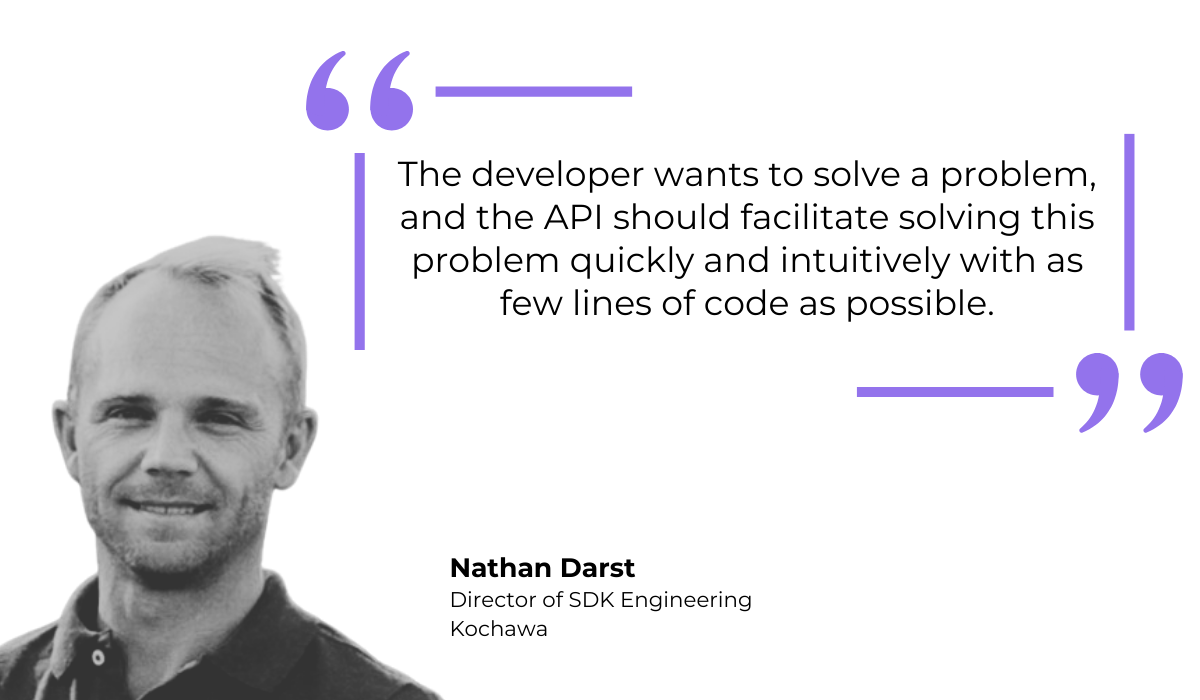
This sentiment highlights how intuitive API design can mean the difference between a seamless integration experience and hours of frustration.
Developers should be able to quickly grasp how to use your SDK with minimal reliance on documentation.
Consider Dropbox, which is renowned for its developer-friendly APIs.
Their API Explorer is a standout feature that makes it easy for developers to understand and interact with the Dropbox API v2.
By simply clicking on an endpoint—a specific URL or method used to access Dropbox’s services—developers can:
- submit API calls,
- view the code for those calls, and
- instantly see the API responses.
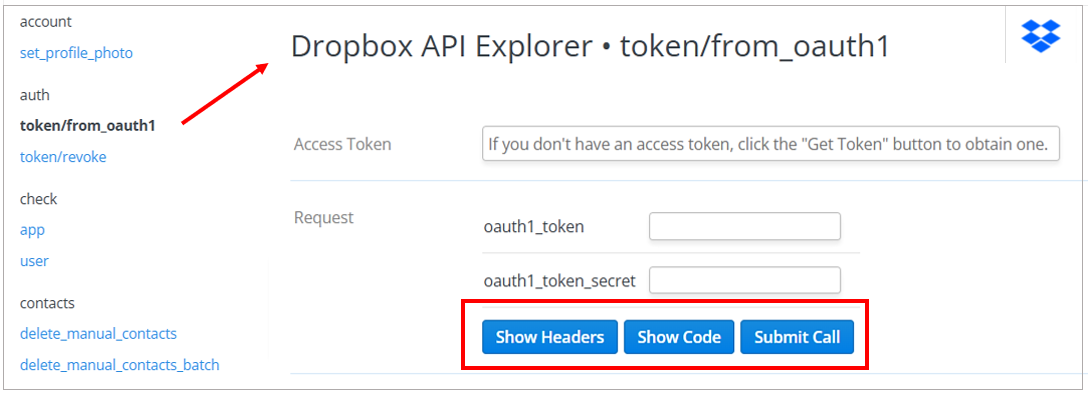
This interactive, hands-on approach reduces complexity, encourages experimentation, and allows developers to learn by doing—key principles of intuitive API design.
To create similarly effective APIs, focus on clarity and usability.
Start with naming conventions by using descriptive, consistent names for API methods, variables, and parameters, avoiding jargon or abbreviations that might confuse users.
Another critical aspect is adhering to patterns—following idiomatic practices for your target language, such as RESTful conventions for web APIs.
Familiar workflows make your SDK easier to adopt and more enjoyable to use.
By prioritizing these principles, you can create APIs that enhance the developer experience and make your SDK a preferred choice for seamless integration.
Ensure robust error handling and debugging
Designing your SDK to include effective error handling and debugging support is essential for delivering a smooth developer experience.
When errors arise, clear and actionable responses help developers quickly identify and fix issues, reducing frustration and saving valuable time during integration and troubleshooting.
A good starting point is crafting meaningful error messages.
Avoid vague descriptions like “Unknown error” or generic error codes, which can leave developers guessing.
Instead, provide specific messages that explain what went wrong and why.
For example, here’s a comparison between an unclear error message (before) and a clearer, actionable alternative, courtesy of UX Planet:
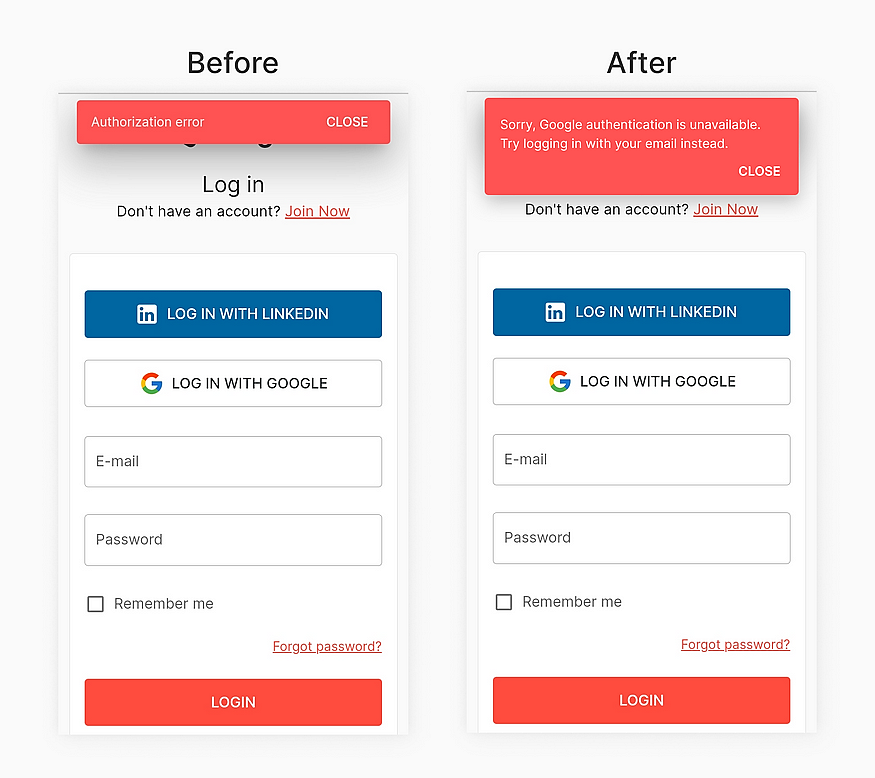
The “before” message is vague because it doesn’t explain why the error occurred.
In this case, developers can only guess whether the issue is related to credentials, the authentication server, or a network problem.
On the other hand, the “after” message is specific and actionable.
It identifies the issue (Google authentication being unavailable) and offers a clear alternative solution (log in with email).
Another best practice is to pair such messages with error codes that link to comprehensive troubleshooting guides in your documentation.
This provides developers with the context they need to resolve issues quickly and efficiently.
Here’s an example of Twitter’s (now X’s) response codes and their explanations:
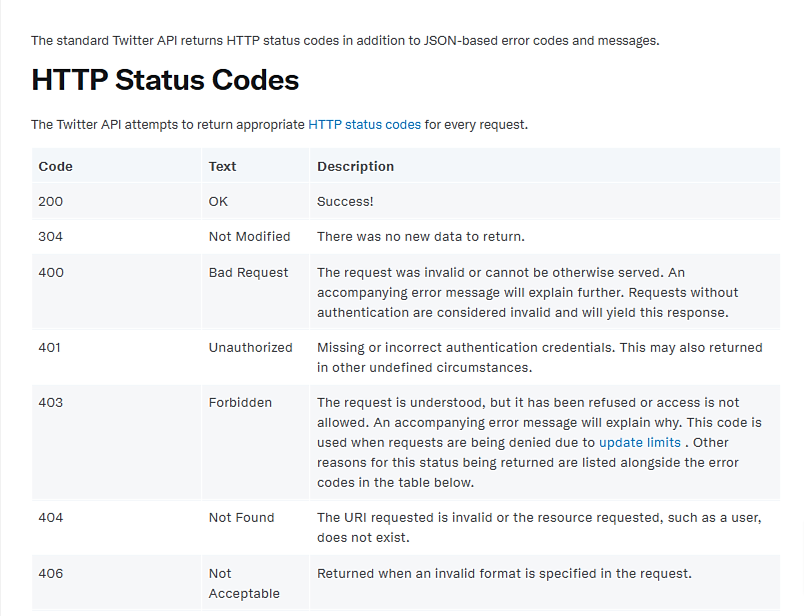
In addition to clear error messages, your SDK should be designed to support robust debugging, enabling developers to quickly diagnose and fix issues.
This can be achieved by providing features such as detailed logs, stack traces, and context-specific error information.
For example, in Zendesk’s Support SDK, developers can enable logging before initializing the SDK, allowing them to capture logs during integration and identify issues easily.
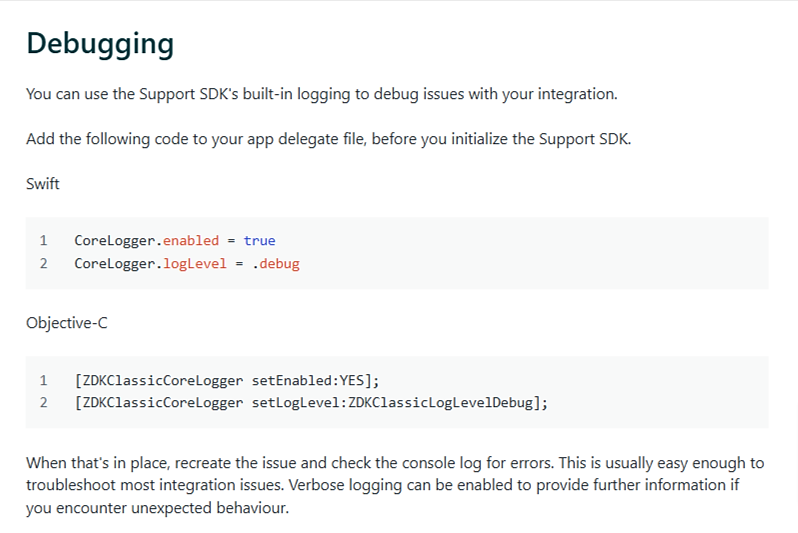
Additionally, implementing different log levels (e.g., debug, info, error) helps in filtering the output based on the severity of messages, making it easier to focus on critical issues.
Another debugging feature is to include information about the environment in which the error occurred, such as device type or operating system version.
When clear error messages and these debugging features are combined, developers are empowered to quickly identify and resolve potential issues with integrating your SDK.
Make the SDK lightweight
A lightweight SDK is essential for seamless integration and optimal performance within a developer’s app.
Overly large or resource-intensive SDKs can bloat app sizes, slow load times, and degrade user experience, discouraging developers from adopting your solution.
To achieve this, include only essential features and avoid unnecessary dependencies.
Adopting a modular architecture allows developers to integrate only the components they need.
For example, Firebase SDK, a comprehensive development tool by Google, enables selective module integration rather than bundling everything by default.
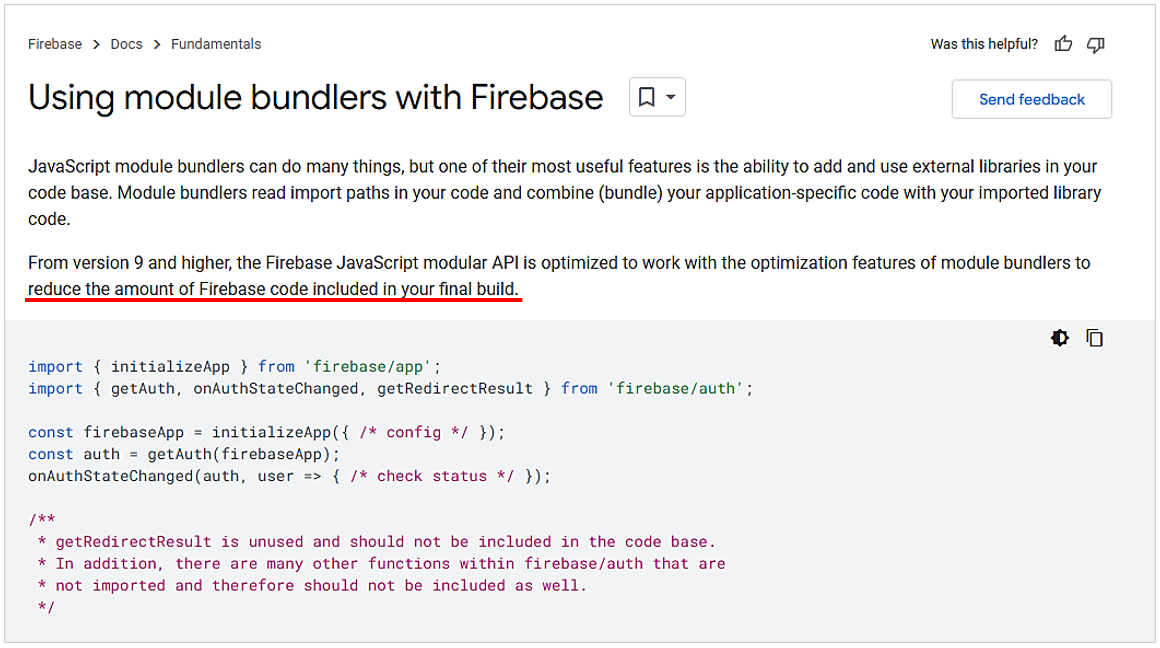
This process of removing unnecessary code from a library is called “tree shaking”.
While doing this manually would be very time-consuming and error-prone, module bundlers, such as Rollup, esbuild, or webpack automate the removal.
Providing clear documentation on your SDK’s size and performance benchmarks is also critical.
It enables developers to understand an SDK’s impact on their app from the outset.
For instance, Shake SDK has a small, 2.5 MB footprint, meaning that it won’t affect your app’s performance, and we communicate this from the outset.
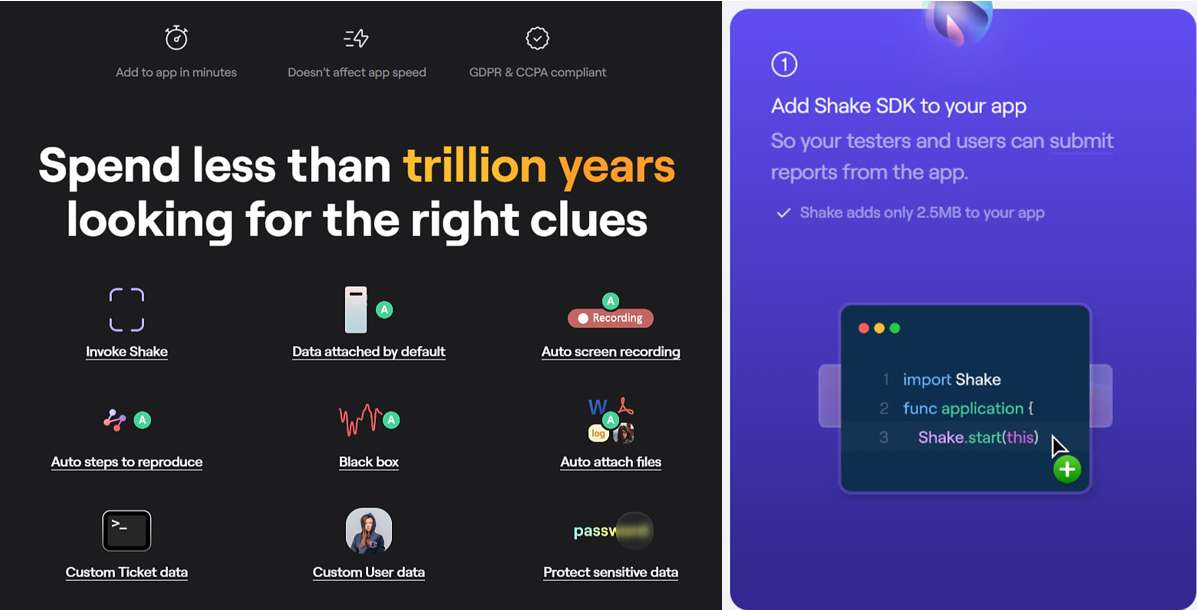
In an environment where app performance and size are critical to user satisfaction, such an SDK will be more appealing to developers.
Therefore, a lightweight SDK positions your product as a reliable, thoughtful choice that respects the constraints of modern app development.
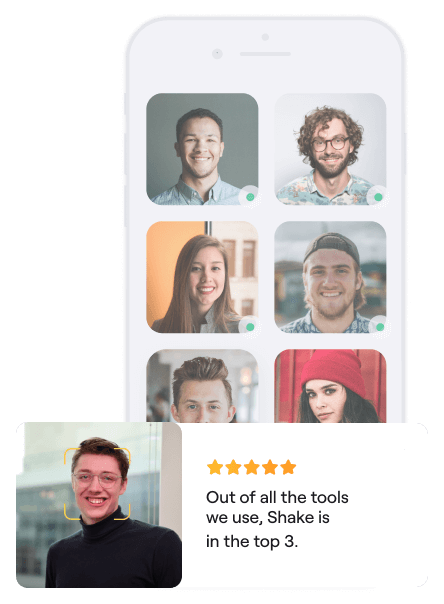
Very handy for handling user feedback. CTOs, devs, testers – rejoice.
Maintain backward compatibility
When updates to an SDK break existing API implementations, developers face unnecessary rework and frustration.
By maintaining backward compatibility, you enable developers to seamlessly adopt new SDK versions without disrupting their workflows.
A widely adopted strategy to achieve this is semantic versioning (SemVer), which uses a three-part versioning scheme to clearly communicate the scope and impact of changes:
- major versions introduce breaking changes that may require developers to modify their code,
- minor versions add new features that are backward-compatible with existing functionality,
- patch versions address bugs without altering any documented behavior.
Here’s an excerpt from Stripe’s SDK versioning policy:

To illustrate, imagine your app integrates with a payment API using Stripe SDK version 4.3.2.
If Stripe releases 4.3.3, it would include a bug fix (patch) that requires no code changes to your integration.
A release of 4.4.0 would introduce a new feature that you could optionally use without altering existing code.
However, 5.0.0 would signal a major version with breaking changes, such as requiring a new parameter in all API calls, which would necessitate modifications to your app’s integration.
This structured versioning minimizes disruptions while ensuring developers are informed about the nature of each update.
Effective deprecation practices are equally critical.
Instead of abruptly removing outdated features, providing clear timelines and comprehensive migration guides helps developers transition smoothly to newer versions.
Microsoft serves as an excellent example in this regard.
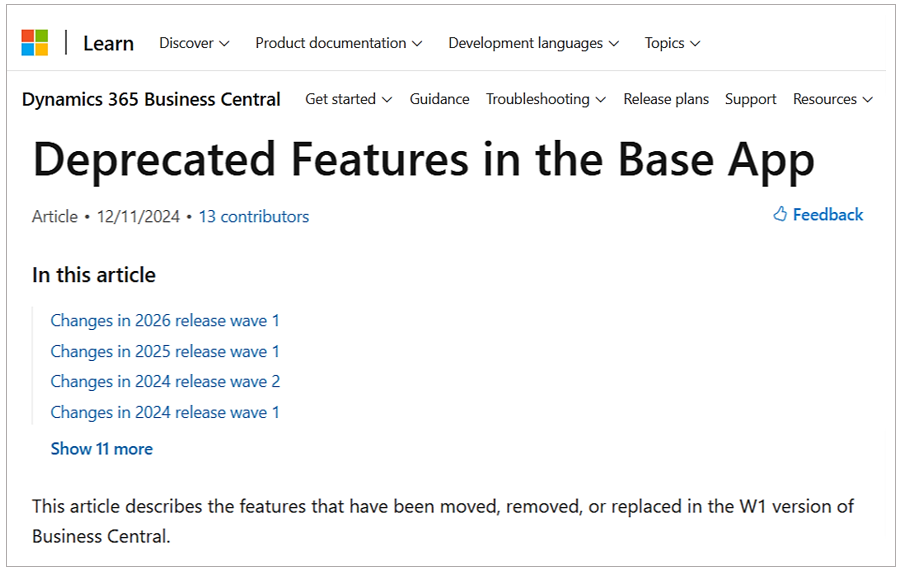
Their documentation consistently highlights deprecated features, both past and upcoming, and provides detailed steps to facilitate transitions to newer implementations.
All in all, clear semantic versioning and robust deprecation practices can ensure long-term backward compatibility maintenance.
In turn, this enhances developer trust and ensures seamless adoption of your SDK updates.
Support your developer community
When creating your SDK, an engaged developer community can foster trust, drive adoption, and provide valuable feedback.
To support this community, ensure you provide clear communication channels, such as dedicated forums, FAQs, and support tickets.
Additionally, it’s crucial to regularly update your documentation and maintain a transparent changelog so developers can stay informed about new features and bug fixes.
We support our users through the documentation on our website but also with our dedicated Slack channel, where users can ask for help directly.
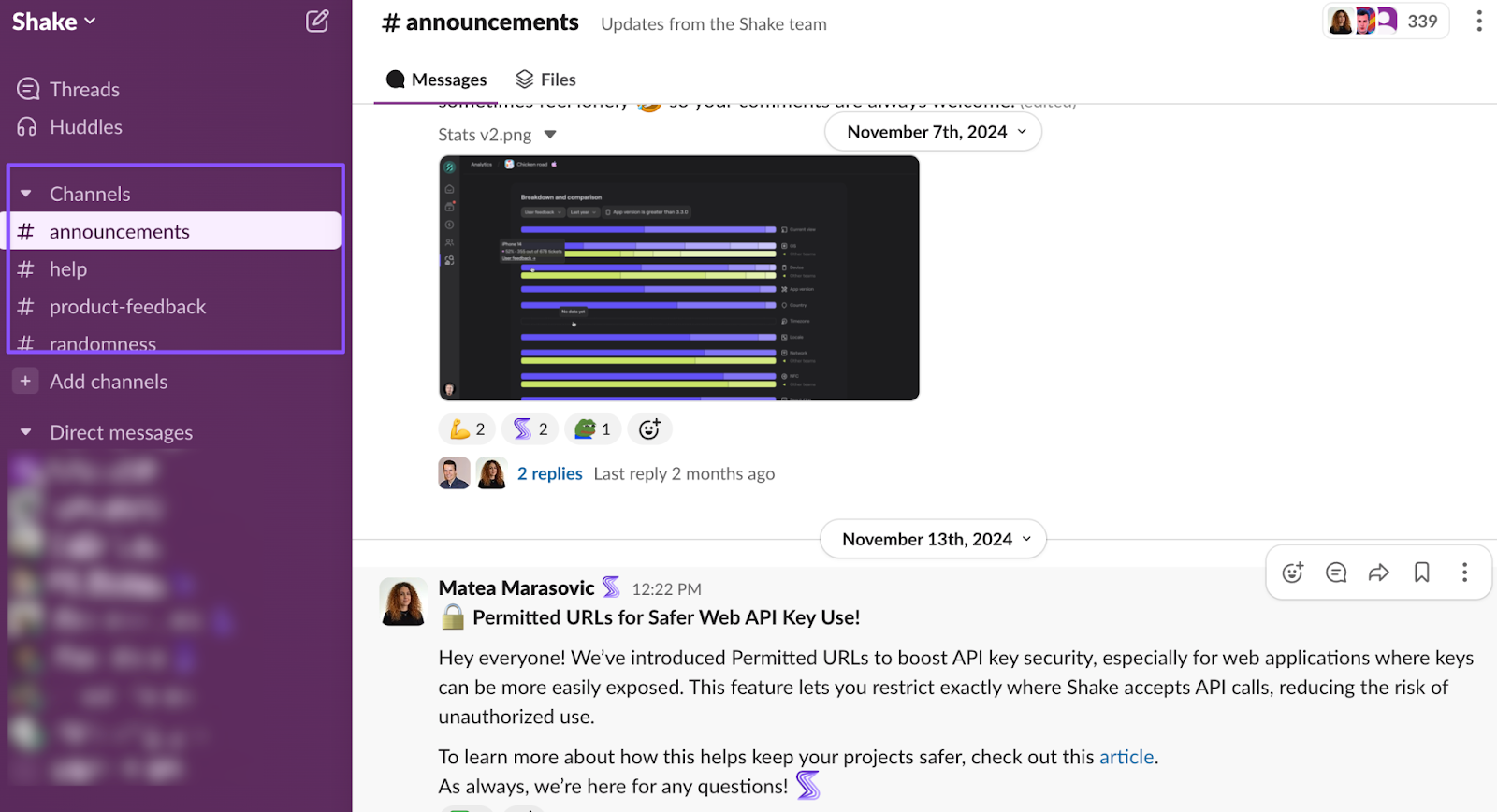
If you are also set on building a strong developer community, you will need to learn how to increase engagement and manage the crowd.
One effective way to do so is by setting up clear contributing guidelines.
For example, GitHub allows project owners to create a special file that outlines how developers can submit pull requests or raise issues effectively.
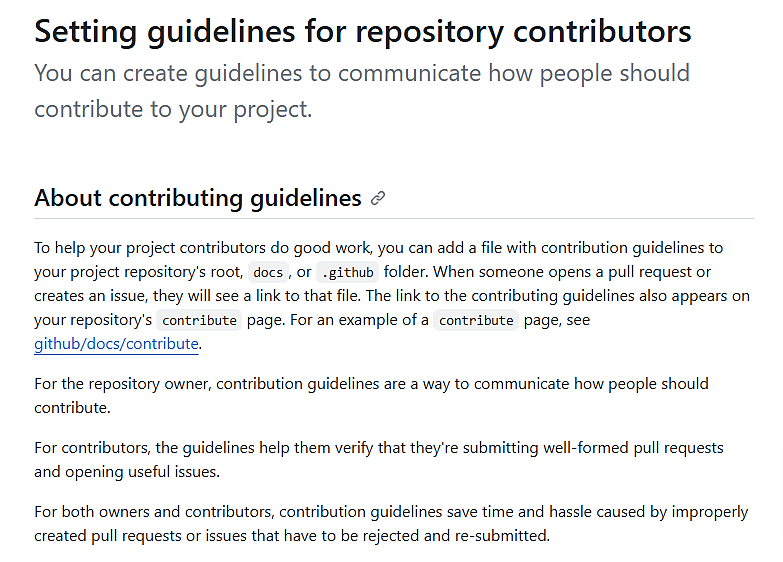
These guidelines help contributors understand how to engage with the project and ensure that their contributions are consistent and useful.
This reduces friction and encourages more developers to contribute to your SDK.
By providing a channel for open communication coupled with clear contribution rules, you can build a collaborative, productive community that strengthens your SDK’s adoption and improvement over time.
Conclusion
Designing an effective SDK involves far more complexity than what this article could fully explore.
However, the best practices outlined here provide a solid foundation for further research and implementation.
Remember, an SDK isn’t just a tool—it’s a partnership with developers.
By investing in its design, you can drive adoption, foster trust, and ensure your SDK becomes an indispensable resource in their journey.